- Understand and plan out a scene flow for a Unity project.
- Understand data persistence
- “Scope/Lifetime” of data (how long should the data last?)
- Storage of data (how is the data stored and read?)
- Understand object persistence
- DontDestroyOnLoad usage.
- Loading and unloading scenes.
- Gain basic exposure to object-oriented programming.
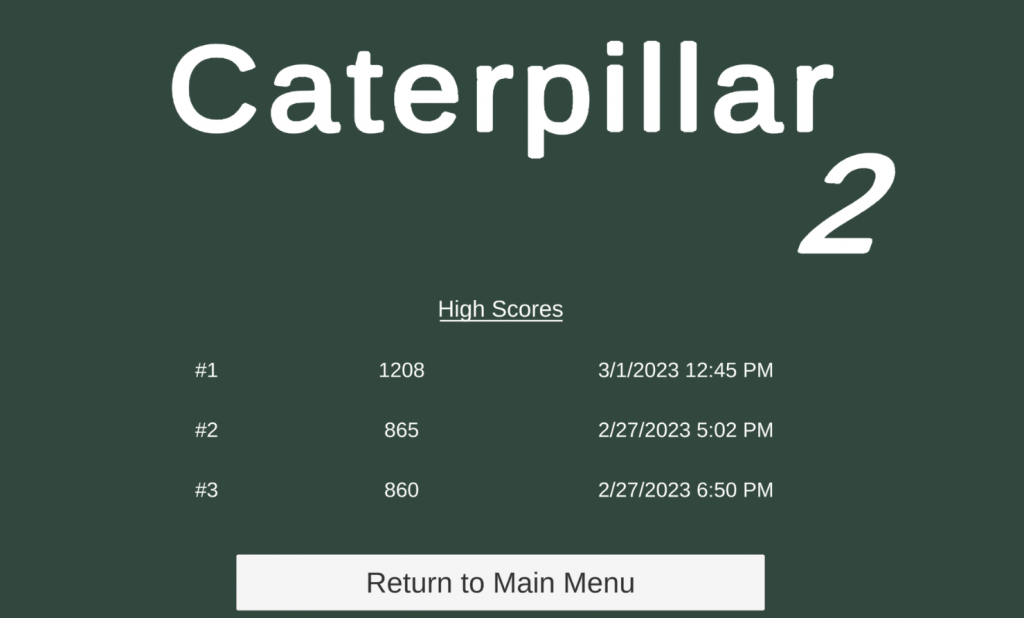
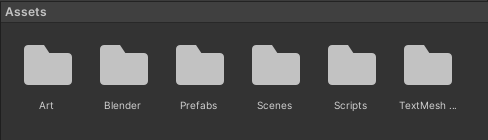
- The game has a new objective. In Caterpillar’s old version, there wasn’t a strong end goal. In this game version, the caterpillar will need to traverse multiple levels. Each level will now have an exit. The objective is to maximize the length of the caterpillar (eat as much food as possible) while also completing the game (making it through each level) in the shortest amount of time.
- There are some new menu scenes! There is now a main menu, settings for the player to change, and a working high scores screen to display achievements.
- A whole slew of new scripts is used to control these new items.
- GameManager makes a return, but as a “singleton” class which makes accessing it easier, as discussed in the Unity learning materials.
- There is now a WorldManager (to control scene changes and levels) and a MenuManager (to control interaction with menu objects)
- Game settings are stored in the “Settings” game object.
- Good Names (so you can keep track of them later)
- A level (floor, four walls, with a “Map” object)
- An exit (any trigger collider with an “Exit” component)
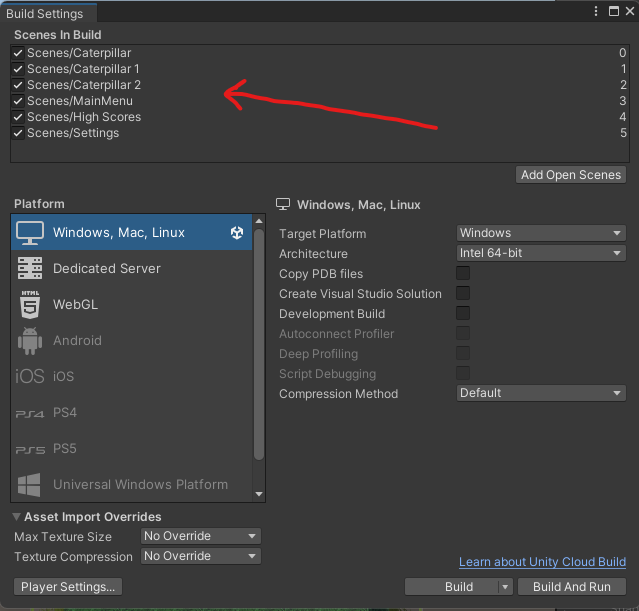
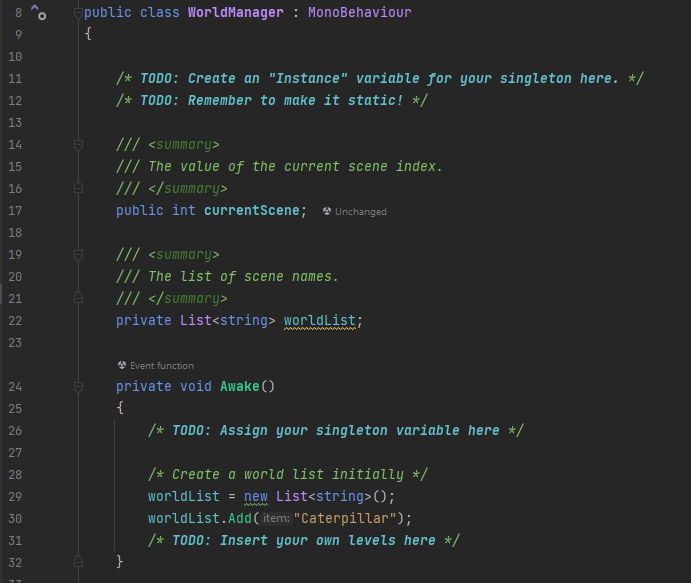
- StartGame()
- StartGame is called when the game is started from the main menu, so it needs to “start the game over.”
- You must add a code line to load in the game’s first level.
- What function is used to load scenes?
- How do you get the name of the first scene?
- NextScene()
- NextScene is called when the caterpillar reaches the exit of a level.
- You need to add a line of code to load the next scene in the list; the level’s name can be found in the nextScene variable.
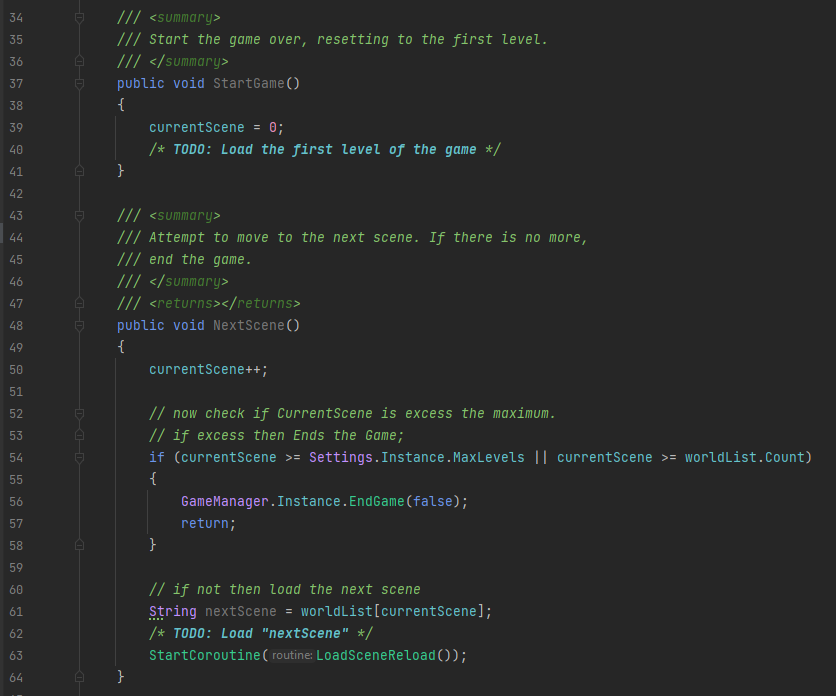
- How do we reference or call functions on the WorldManager when it has a static “Instance” variable?
- How do we use a conditional to tell what type of object we ran into?
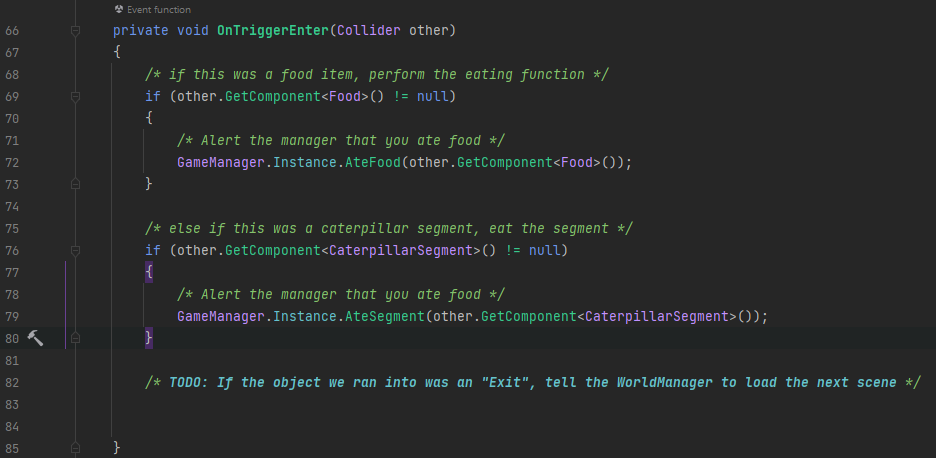
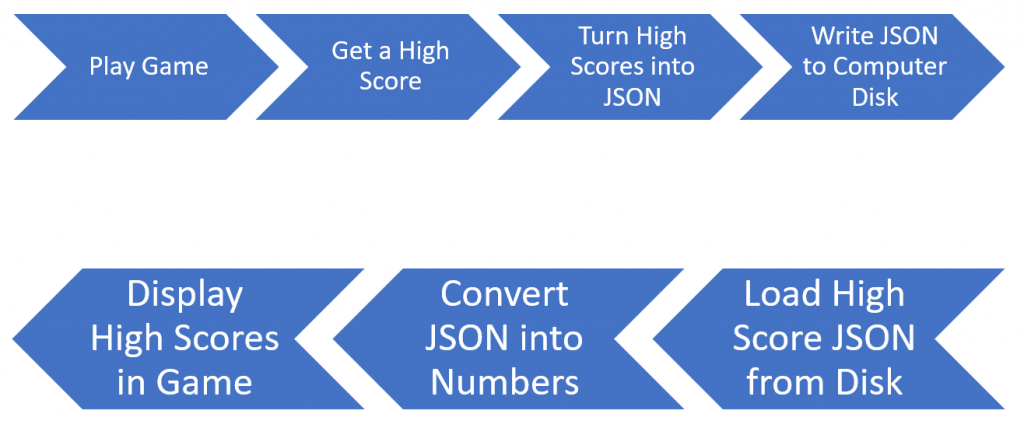
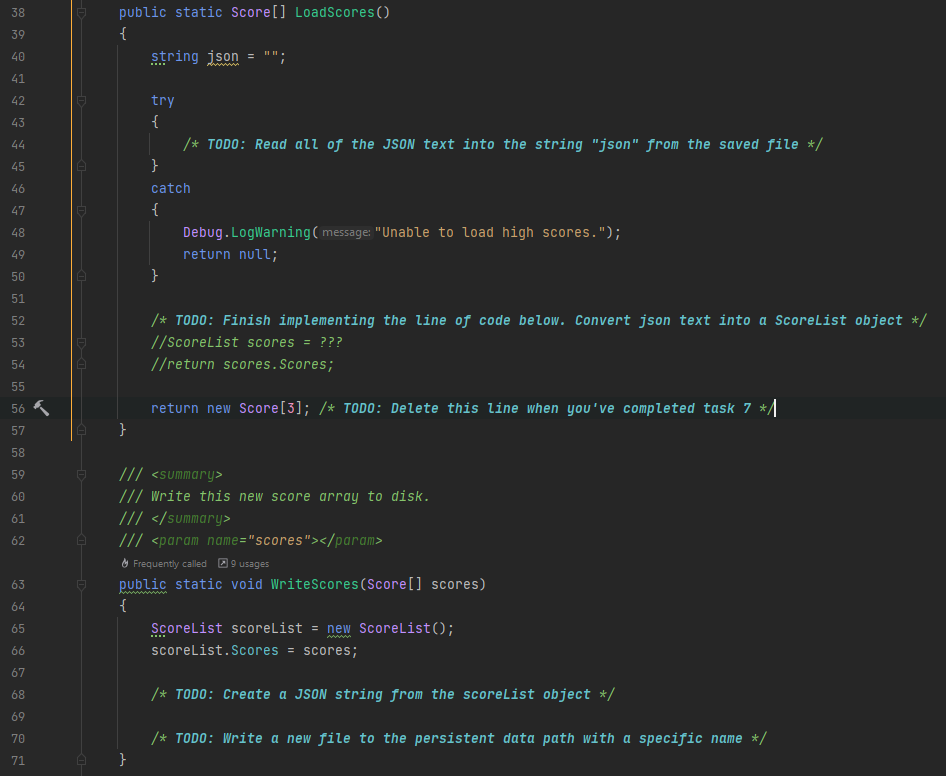
- Data structures (singletons, instance variables, static variables).
- Scene loading and scene flow.
- Data persistence (keeping data around after a scene is unloaded or when the game is closed).