- Prototyping and fast iteration of a Unity game
- Selecting appropriate data types for required functionality
- Utilize the Unity API in order to use Unity’s advanced features
- Write code in a readable, efficient style
- Debug code at a basic level
- Compile Errors
- Runtime Errors (exceptions)
- Logic Errors
- Logic Structures
- Lists
- “for” loops
- Control flow
- Effects
- Sounds
- Particle Effects
- Unit 3 – Sound and Effects
- Unit 4 – Gameplay Mechanics
- Unit 5 – User Interface
- Introduction to user feedback and testing
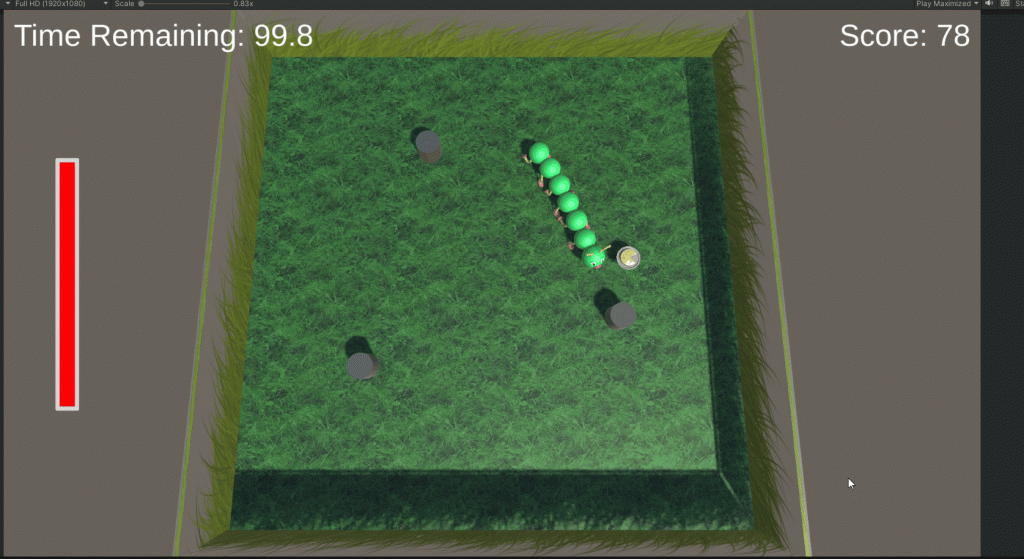
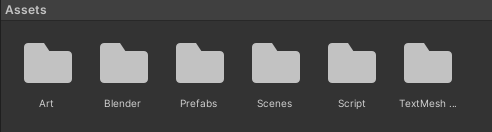
- The floor (a plane) should be of scale (10,10,10) and positioned at (0,0,0).
- Each side of the plane (+x,-x,+z,-z) should have a wall (made from a stretched cube).
- You should place a few obstacles (rocks are located in Assets > Art > Models) around the map to add difficulty to your game.
- All map components (floor, walls, obstacles) should be children of the “Map” game object.
- Create a new material for the floor, the walls, and the obstacles.
- Apply the materials to the floor, walls, and obstacles.
- Add an image texture to at least one of the materials.
- Alter the “tiling” on the image texture’s material to change the look of the texture.
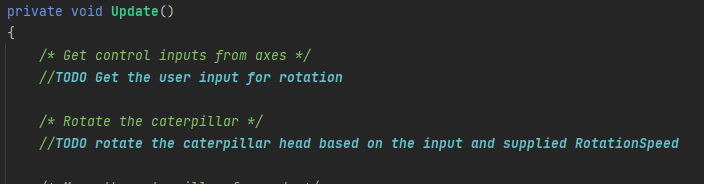
- For each segment in the segments list (excluding the first)
- Save the “direction” from each segment to the segment before it in the array
- Move the segment slightly in the saved direction
- Rotate the segment to more closely match the direction it moved
- Move the first segment towards the head of the caterpillar
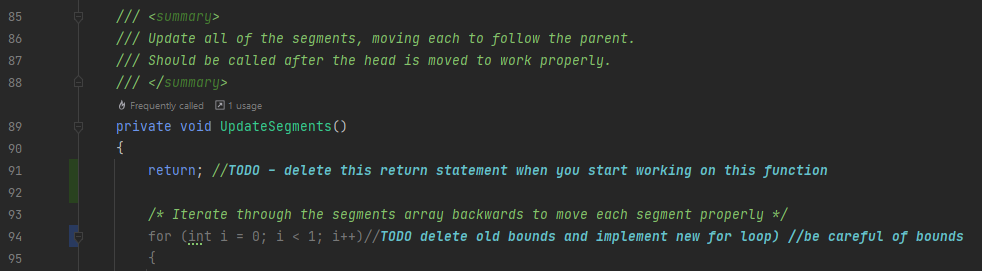
- If the item collided with has a “Food” component, call the “AteFood” function on the game manager component (you’ll have to “locate” the game manager somehow)
- If the item collided with has a “CaterpillarSegment” component, call the “AteSegment” function on the game manager component.
- How can you see if a component is present on a supplied object?
- How can you get a reference to a type of game object in the scene (hint: look up “Find” functions).
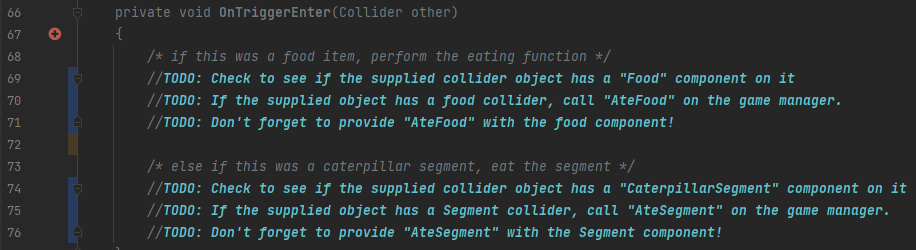
- Find the index of the segment which got eaten.
- Compute (and store) the segments which need to be removed.
- How do we know which segments need to be removed? If the caterpillar has 10 segments and the 5th is eaten, all segments between the 5th and the 10th (inclusive) should be removed (they “fall off”).
- Remove the segments which need to be removed.
- Remove the segments from the segments list, not the “segmentsRemoved” list
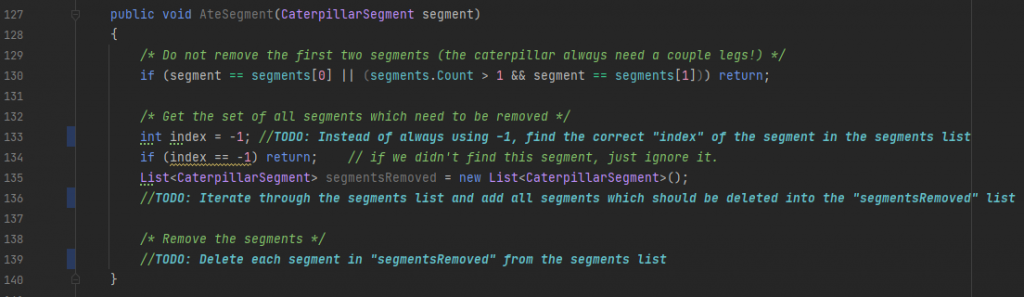
- Move the segment into the correct position (it should match the position of the last segment on the caterpillar).
- Add the new segment into the segments list.
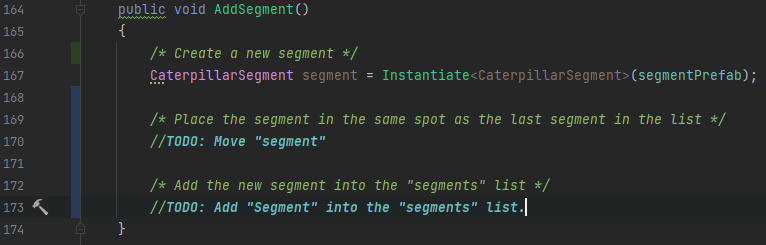
- Are you happy with the forward speed of the caterpillar? What about the turning speed?
- Do you like how score is calculated? How might you want to change it?
- Is there too much time in the level? Too little time?
- What graphics look bad to you? Can you replace them? What about color scheme?
- There’s no sound currently. How might you implement sound?