- Install the Unity editor and create new projects
- Navigate and utilize the Unity editor
- Create and edit scenes
- Add primitive objects
- Translate, rotate, and scale objects within the editor
- Add components to gameobjects
- Prefabs
- Physics systems
- Create interaction with basic C# scripting
- GameObjects, Transforms, and Vectors
- Variables
- Start and Update events
- IDE literacy
- Import and apply visual and auditory effects
- Materials
- Importing 3D models
- Sound effects
- Mission 1: Get Started with Unity
- Mission 2: Explore Unity
- Mission 3: Learn Unity
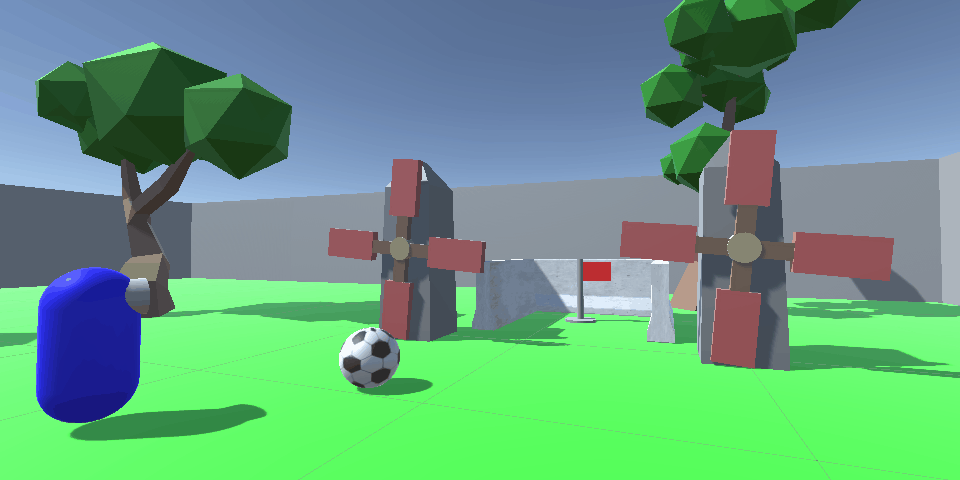
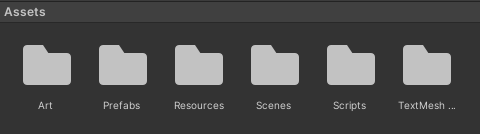
- Player (the thing you move around)
- Hole/Flag (the flag which you need to kick the ball into)
- Ball (the soccer ball you kick around)
- Trees
- Bushes
- Windmills
- Barriers
- Does your level have a player, ball, and hole/flag?
- Do you have some obstacles in your level? (Trees, bushes, walls, windmills)
- Did you adjust your color scheme?
- Edit the properties on the “Player” component on the Player gameObject. Should the player move faster, turn faster, or kick harder? This is up to you.
- Edit the properties on the Ball’s rigidbody object. Should the ball have more or less friction? Should the ball be heavier?
- Edit colors and materials. Is anything hard to see? Do you want to change the color scheme of the game?
- Do you have a windmill? Maybe the blades should move faster, or slower, depending on the level you made.
- Is your level too hard? Too easy? Move, add, or remove obstacles to change things up!